2.7 内存对齐
1. 获取变量占用的内存大小
Golang 中可以使用 unsafe.Sizeof
获取变量的内存占用。
type Args struct {
num1 int
num2 int
}
type Flag struct {
num1 int16
num2 int32
}
func main() {
fmt.Println(unsafe.Sizeof(Args{}))
fmt.Println(unsafe.Sizeof(Flag{}))
}
// output
16
8
Args
:有两个 int 型字段,在 64 位系统中占用 8 ByteFlag
:内存占用理论上是 4+2=6,结果为 8 Byte,多出的 2 Byte 是内存对齐的结果
故一个变量的内存占用包含:
- 各字段占用空间之和
- 内存对齐补充的空间
2. 内存对齐
2.1 为什么需要内存对齐
CPU 访问内存时,不是逐个字节的访问,而是以字长(word size)为单位访问。如32位系统的字长为 4 字节。
采用字长可以减少 CPU 访问内存的次数,增大 CPU 访问内存的吞吐量。
若不进行内存对齐,会增大 CPU 的访问次数:
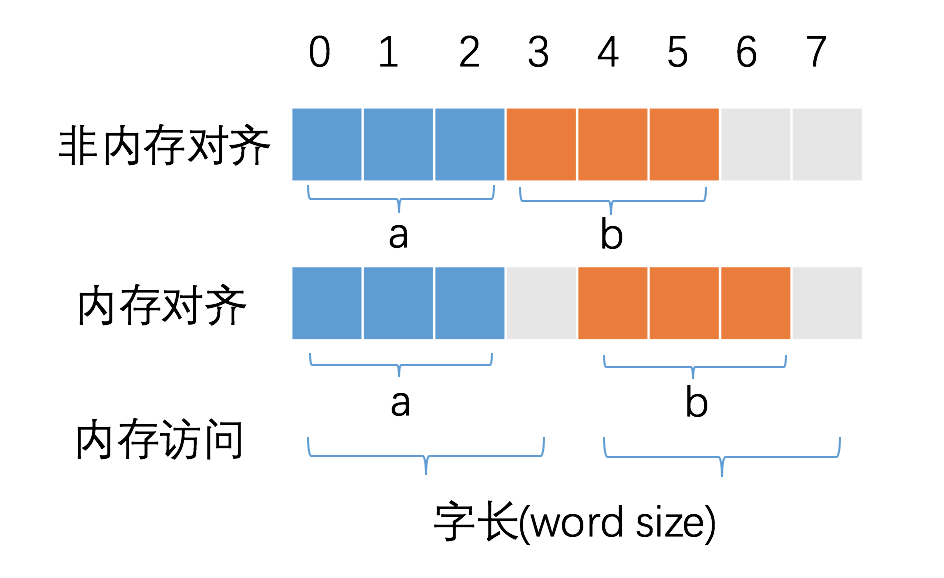
由上图所示,a 和 b 各占用 3 字节,当CPU想要读取 b 的值时:
- 若不进行内存对齐,需要读取两次,第一次获取 b 的第一个字节,第二次获取剩余的两个字节
- 若进行内存对齐,只需读取一次内存即可
若变量的内存占用不超过字长时,在内存对齐的情况下,CPU 读取变量是原子性的。
2.2 unsafe.Alignof
unsafe.Alignof
返回类型的对齐系数,那么此类型的变量占用的内存就必须是对齐系数的倍数。
unsafe.Alignof(Args{}) // 8
unsafe.Alignof(Flag{}) // 4
Args{}
的对齐倍数是 8,Args{}
两个字段占据 16 字节,是 8 的倍数,无需占据额外的空间对齐。Flag{}
的对齐倍数是 4,因此Flag{}
占据的空间必须是 4 的倍数,因此,6 内存对齐后是 8 字节。
2.3 对齐保证(align guarantee)
Golang 官方文档 Size and alignment guarantees - golang spec 描述了函数unsafe.Alignof
的规则:
- For a variable x of any type: unsafe.Alignof(x) is at least 1.
- For a variable x of struct type: unsafe.Alignof(x) is the largest of all the values unsafe.Alignof(x.f) for each field f of x, but at least 1.
- For a variable x of array type: unsafe.Alignof(x) is the same as the alignment of a variable of the array’s element type.
- 对于任意类型的变量 x ,
unsafe.Alignof(x)
至少为 1。 - 对于 struct 结构体类型的变量 x,计算 x 每一个字段 f 的
unsafe.Alignof(x.f)
,unsafe.Alignof(x)
等于其中的最大值。 - 对于 array 数组类型的变量 x,
unsafe.Alignof(x)
等于构成数组的元素类型的对齐倍数。
A struct or array type has size zero if it contains no fields (or elements, respectively) that have a size greater than zero. Two distinct zero-size variables may have the same address in memory.
没有任何字段的空 struct{} 和没有任何元素的 array 占据的内存空间大小为 0,不同的大小为 0 的变量可能指向同一块地址。
3. 内存对齐技巧
3.1 合理布局减少内存占用
假设一个 struct 包含三个字段,a int8
、b int16
、c int64
:
type demo1 struct {
a int8
b int16
c int32
}
type demo2 struct {
a int8
c int32
b int16
}
func main() {
fmt.Println(unsafe.Sizeof(demo1{})) // 8
fmt.Println(unsafe.Sizeof(demo2{})) // 12
}
可以看出结构体中的字段顺序会对结构整体的内存占用产生影响。
每个字段会根据自己的对齐系数来计算内存中的偏移量,字段排列顺序不同,前一个字段因偏移而浪费的内存大小不同。
对于 demo1
,结构体的对齐系数会是字段中最大的那个 :
a int8
,对齐系数为 1,已对齐b int16
,对齐系数为 2,整体占用 3 = 1+2,需要空出一个字节进行对齐c int32
,对齐系数为 4,整体占用 8= 1+1+2+4,已对齐
对于demo2
:
a int8
:对齐系数为 1,已对齐b int32
:对齐系数为 4,整体占用 5 = 1+4,需空出 3 个字节进行对齐c int16
:对齐系数为 2,结构体对齐系数为 4,整体占用 10 = 1+3+4+2,需空出 2 个字节进行对齐
demo2
对齐后的内存占用为:12 = 1+3+4+2+2
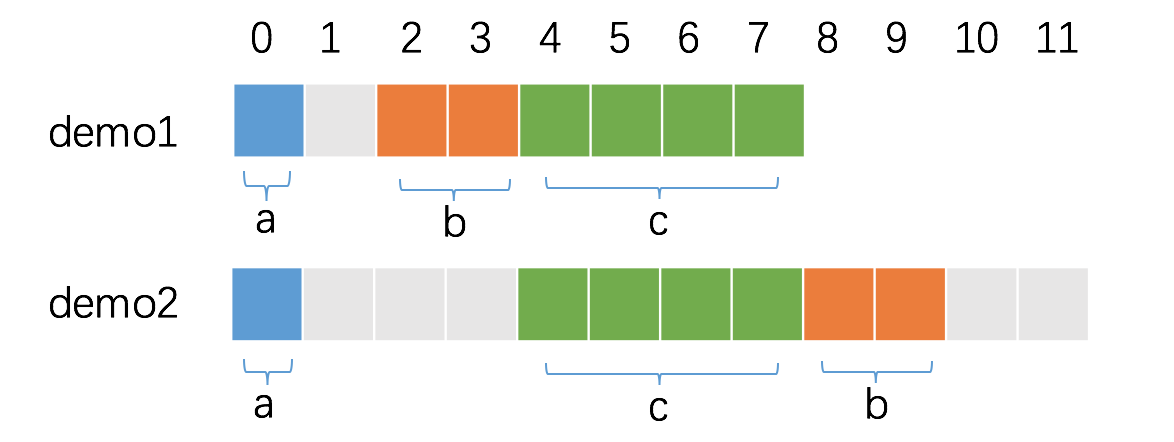
因此在内存敏感的结构体设计上,可以通过调整字段顺序,减少内存占用。
3.2 空结构体的内存对齐
空struct{}
大小为 0,作为 struct 的字段时,一般无需对齐。
但是若 struct{}
作为最后一个字段时,需要进行内存对齐。因为如果有指针指向该字段,返回的地址将会在结构体之外,如果此指针一直存活不释放对应的内存,就会有内存泄露的问题(该内存不因结构体释放而释放)。
type demo3 struct {
c int32
a struct{}
}
type demo4 struct {
a struct{}
c int32
}
func main() {
fmt.Println(unsafe.Sizeof(demo3{})) // 8
fmt.Println(unsafe.Sizeof(demo4{})) // 4
}
demo3
中:
c int32
:对齐系数为 4,整体系数为 4 ,占用 4 B,无需对齐a struct{}
:虽然内存占用为0,但是需要额外的内存对齐,对齐 4 字节